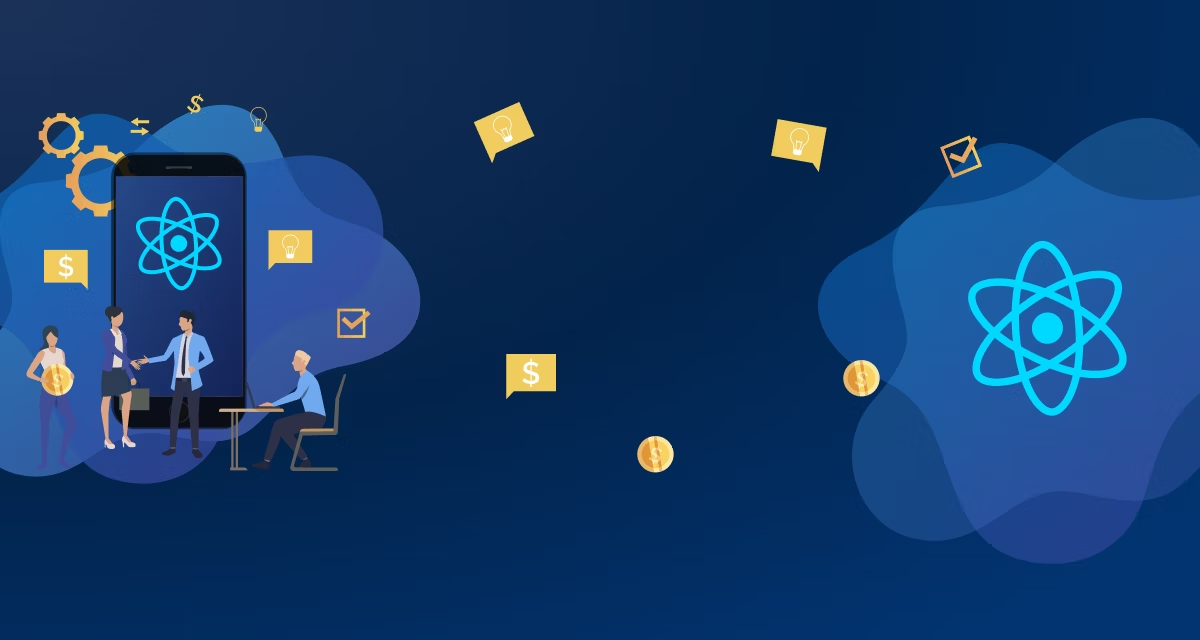
The Complete React.js Roadmap: Mastering the Fundamentals to Advanced Concepts
Introduction
Welcome to the comprehensive React.js roadmap! Whether you're a beginner or an experienced developer looking to enhance your React skills, this guide will take you through the essential concepts and advanced topics to become a React pro.
Components
React revolves around components, building blocks of UI. Understand the basics:
- Functional Components: UI with functions.
- Class Components: The traditional class-based approach.
- JSX (JavaScript XML) Syntax: Declarative UI syntax.
Props(Properties)
Learn how to handle component properties:
- Passing Props: Communicating between components.
- Default Props: Providing default values.
- Prop Types: Type-checking for props.
State
Master state management techniques:
- useState Hook: Managing state in functional components.
- Class Component State: Understand state in class components.
- Immutable State: Ensure consistency through immutability.
Lifecycle Methods
Gain insight into the lifecycle of React components:
- componentDidMount: Initialize and set up components.
- componentDidUpdate: Manage updates and re-rendering.
- componentWillUnmount: Execute cleanup operations when components are unmounted.
Hooks
Discover the versatility of React hooks:
- useState
- useEffect
- useContext
- useReducer
- useCallback
- useMemo
- useRef
- useImperativeHandle
- useLayoutEffect
Event Handling
Learn to handle events proficiently in both functional and class components.
Conditional Rendering
Dive into rendering techniques based on conditions:
- if Statements
- Ternary Operators
- Logical && Operator
Lists and Keys
Efficiently render dynamic lists and manage keys for optimal performance.
Component Composition
Master the art of component composition:
- Reusing Components
- Children Props
- Composition vs. Inheritance
Higher-Order Components (HOC)
Explore the concept of HOCs to encapsulate and reuse component logic effectively.
Render Props
Unlock flexibility in component rendering using the Render Props pattern.
React Router
Effortlessly navigate your React application with:
- BrowserRouter
- Route
- Link
- Switch
- Route Parameters
Navigation
Streamline navigation using hooks:
- useHistory Hook
- useLocation Hook
State Management
Explore various state management options:
- Context API: Create and use context for global state.
- Redux: Dive into Actions, Reducers, Store, and React-Redux integration.
Forms
Efficiently handle form data:
- Controlled Components
- Uncontrolled Components
Side Effects
Effectively manage side effects using the useEffect hook.
AJAX Requests
Fetch data seamlessly with popular libraries:
- Fetch API
- Axios Library
Error Handling
Gracefully handle errors with:
- Error Boundaries
- componentDidCatch (Class Components)
- ErrorBoundary Component (Functional Components)
Testing
Ensure code reliability through testing:
- Jest Testing Framework
- React Testing Library
Best Practices
Adopt industry best practices:
- Code Splitting
- PureComponent and React.memo
- Avoiding Reconciliation
- Keys for Dynamic Lists
Optimization
- Memoization
- Profiling and Performance Monitoring
Build and Deployment
Prepare your application for production:
- Create React App (CRA)
- Production Builds
- Deployment Strategies
Frameworks and Libraries
Explore popular React libraries:
- Styling Libraries:Styled-components, CSS Modules
- State Management Libraries:Redux, MobX
- Routing Libraries: React Router, Reach Router
React Resources
- Documentation
- YouTube Channels
Conclusion
Congratulations on completing this comprehensive React.js roadmap! You've embarked on a journey from the foundational concepts of React.js to mastering advanced techniques, equipping yourself with the skills needed to build dynamic and efficient user interfaces.
As you reflect on your journey, remember that React is a powerful library that constantly evolves. Stay curious, keep exploring the latest updates, and continue honing your skills. The roadmap has covered a diverse range of topics, ensuring you have a solid understanding of React's core principles, state management, routing, and best practices.
In your development endeavors, always consider the broader ecosystem. Leverage styling libraries like Styled-components, adopt state management solutions such as Redux or MobX, and optimize your applications for superior performance.
Don't forget the importance of testing and following best practices, as these contribute to the reliability and maintainability of your code. Whether you're building small projects or large-scale applications, the principles you've learned here will serve as a solid foundation.
Lastly, utilize the provided resources, including the official documentation and recommended YouTube channels, to stay updated and connected with the vibrant React community. Continue learning, collaborating, and pushing the boundaries of what you can create with React.js.
Your journey with React doesn't end here; it's a continuous adventure of growth and innovation. Best of luck on your React.js endeavors, and may your coding adventures be both challenging and rewarding!